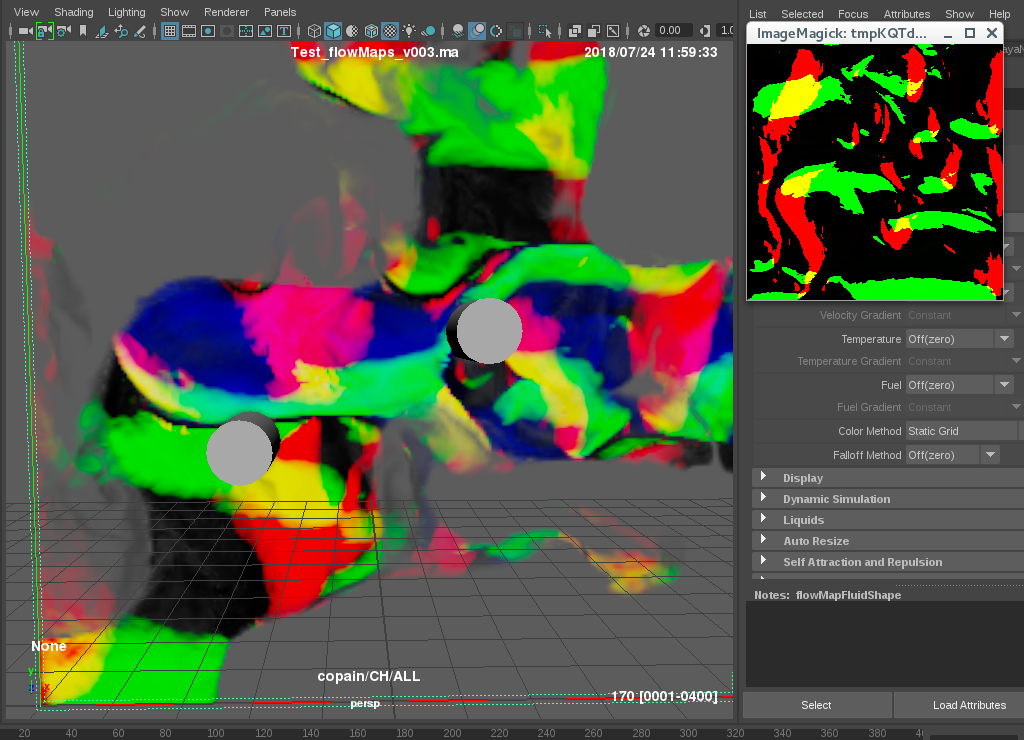
Hi there, so I've hit a little snag and I'm wondering if anyone in the community can help me with this problem.
I'm trying to generate flow maps from a maya fluid. I'm passing velocity data to the R,G channels of an image. Problem is no matter how I do this the end color values are "burnt out". There's no gradiation. (The values are also inverted) When I sample the colors I get either [0,0,0] or [255,0,0] and nothing in between. I've tried changing the data type, but PIL doesn't like anything except uint8 for this type of function. Ideally I want to get an image that has positive and negative color values.
Here's a copy of the code I'm using
import maya.cmds as cmd
from PIL import Image
import numpy as np
fluid = 'flowMapFluidShape'
res = cmd.getAttr('%s.baseResolution' % fluid)
fludArr = cmd.getAttr('%s.fieldData.fieldDataVelocity' % fluid)
massArr = cmd.getAttr('%s.fieldData.fieldDataMass ' % fluid)
#create a 2D array of the same size as the fluid
c_array = np.zeros([res, res, 3],dtype = np.uint8)
#fill the array with velocity and mass data
i=0
for y in reversed(range (res)):
for x in range (res):
c_array[y, x] = [fludArr[i][1], fludArr[i][0], massArr[i]]
#visualise the fluid in viewport
#cmd.setFluidAttr(fluid, at='color', vv = [(fludArr[i][1] ), (fludArr[i][0]), (massArr[i])], xi=x, yi=y, zi=0 )
i+=1
img = Image.fromarray(c_array, 'RGB')
img.save('my.png')
img.show()
help(Image.fromarray)